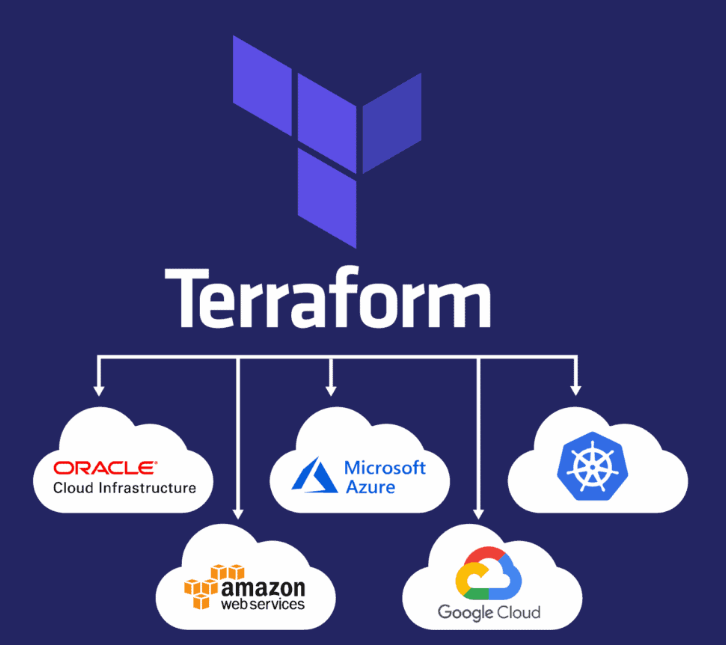
This example provisions a Virtual Machine with Managed Disks. Other examples of the azurerm_virtual_machine
resource , here we will discuss more on the main.tf, variabels.tf wrt azure vm
Variables.tf
# variables.tf
variable "resource_group_name" {
type = string
description = "The name of the Azure resource group."
}
variable "location" {
type = string
description = "The Azure region where resources will be provisioned."
}
variable "vm_name" {
type = string
description = "The name of the Azure VM."
}
variable "vm_size" {
type = string
description = "The size of the Azure VM, e.g., Standard_DS2_v2."
default = "Standard_DS2_v2"
}
variable "admin_username" {
type = string
description = "The username for the VM."
}
variable "admin_password" {
type = string
description = "The password for the VM."
}
variable "os_disk_size_gb" {
type = number
description = "The size of the OS disk in gigabytes."
default = 30
}
variable "network_security_group" {
type = string
description = "The name of the Network Security Group associated with the VM."
}
variable "virtual_network" {
type = string
description = "The name of the Virtual Network associated with the VM."
}
variable "subnet" {
type = string
description = "The name of the Subnet associated with the VM."
}
main.tf
# main.tf
provider "azurerm" {
features = {}
}
resource "azurerm_resource_group" "example" {
name = var.resource_group_name
location = var.location
}
resource "azurerm_virtual_network" "example" {
name = var.virtual_network
address_space = ["10.0.0.0/16"]
location = azurerm_resource_group.example.location
resource_group_name = azurerm_resource_group.example.name
}
resource "azurerm_subnet" "example" {
name = var.subnet
resource_group_name = azurerm_resource_group.example.name
virtual_network_name = azurerm_virtual_network.example.name
address_prefixes = ["10.0.1.0/24"]
}
resource "azurerm_network_security_group" "example" {
name = var.network_security_group
location = azurerm_resource_group.example.location
resource_group_name = azurerm_resource_group.example.name
}
resource "azurerm_network_interface" "example" {
name = "${var.vm_name}-nic"
location = azurerm_resource_group.example.location
resource_group_name = azurerm_resource_group.example.name
ip_configuration {
name = "internal"
subnet_id = azurerm_subnet.example.id
private_ip_address_allocation = "Dynamic"
}
}
resource "azurerm_windows_virtual_machine" "example" {
name = var.vm_name
resource_group_name = azurerm_resource_group.example.name
location = azurerm_resource_group.example.location
size = var.vm_size
admin_username = var.admin_username
admin_password = var.admin_password
network_interface_ids = [azurerm_network_interface.example.id]
os_disk {
caching = "ReadWrite"
storage_account_type = "Standard_LRS"
disk_size_gb = var.os_disk_size_gb
}
source_image_reference {
publisher = "MicrosoftWindowsServer"
offer = "WindowsServer"
sku = "2019-Datacenter"
version = "latest"
}
provision_vm_agent = true
}
dev.tfvars
# terraform.tfvars
resource_group_name = "example-rg"
location = "East US"
vm_name = "example-vm"
admin_username = "adminuser"
admin_password = "P@ssw0rd123!"
network_security_group = "example-nsg"
virtual_network = "example-vnet"
subnet = "example-subnet"
How to use the tfvars in terraform plan & apply
terraform plan -var-file=path/to/your/variables.tfvars
terraform apply -var-file=path/to/your/variables.tfvars