In Ansible, you can use loops to iterate over a set of values and perform tasks repeatedly. Here’s an example of an Ansible playbook that uses a loop
---
- name: Deploy Software with Loop
hosts: all
become: yes
vars:
software_packages:
- nginx
- apache2
- mysql-server
tasks:
- name: Install Software Packages
package:
name: "{{ item }}"
state: present
with_items: "{{ software_packages }}"
hosts
: Specifies the target hosts or group of hosts on which the tasks will be executedbecome
: Used to execute tasks with elevated privileges (sudo)vars
: Defines a variable namedsoftware_packages
that contains a list of software packages to be installedtasks
: Contains a list of tasks to be executed- Install Software Packages: Uses the
package
Ansible module to install software packages. Thewith_items
parameter is used to loop over thesoftware_packages
list
In this example, the playbook installs Nginx, Apache2, and MySQL Server on the target servers. You can customize the software_packages
list with the names of the software packages you want to deploy.
Make sure to replace all with the appropriate group or host in your inventory file. Adjust the package names or add more packages to the software_packages
list based on your requirements.
An example Ansible playbook named ‘Example Playbook’ designed to operate on all hosts with elevated privileges. The playbook includes tasks to stop the Nginx service, uninstall Nginx, and ensure that Nginx is installed, showcasing a series of actions related to the Nginx web server.
---
- name: Example Playbook
hosts: all
become: yes
tasks:
- name: stop nginx
service:
name: nginx
state: stopped
- name: uninstall nginx
apt: name=nginx state=absent
- name: Ensure Nginx is installed
apt:
name: nginx
state: present
“Implementing an Ansible playbook to deploy Git and Python onto remote machines, incorporating loops for efficient task iteration and conditions to ensure controlled deployment.”
- name: install multiple softwares
hosts: all
tasks:
- name: install softwares multiple
apt:
name: "{{ item }}"
state: latest
when: (ansible_facts['distribution'] == 'Ubuntu')
loop:
- git
- python3
Ansible playbook named ‘install multiple softwares’ that targets all hosts, deploying Git and Python 3 with the ‘apt’ module. The installation is conditioned on the host’s distribution being Ubuntu, ensuring the latest versions of the software are installed
you can use the local-exec
provisioner in Terraform. Below is an example of how you might structure your Terraform configuration
resource "null_resource" "execute_ansible_playbook" {
triggers = {
always_run = "${timestamp()}"
}
provisioner "local-exec" {
command = "ansible-playbook -i inventory.ini playbook.yml"
}
}
Actual Exectuion in our Terraform Script using local-exec
resource "null_resource" "remoteExecProvisioner" {
triggers = {
always_run = "${timestamp()}"
}
provisioner "local-exec" {
#command = "ansible all -m shell -a 'apt -y install apache2' -u adminuser"
command = "ansible-playbook './ansible-playbook-with-items.yaml' -u adminuser"
}
Local Exec in the main.tf
terraform {
required_version = ">= 1.2.2"
required_providers {
azurerm = {
source = "hashicorp/azurerm"
version = ">= 3.10.0"
}
}
}
provider "azurerm" {
features {}
}
data "azurerm_resource_group" "example" {
name = "8pm-RG"
}
resource "azurerm_virtual_network" "example" {
name = "myVnet"
address_space = ["10.0.0.0/16"]
location = "Southeast Asia"
resource_group_name = data.azurerm_resource_group.example.name
}
resource "azurerm_subnet" "example" {
name = "mySubnet"
resource_group_name = data.azurerm_resource_group.example.name
virtual_network_name = azurerm_virtual_network.example.name
address_prefixes = ["10.0.1.0/24"]
}
resource "azurerm_network_security_group" "example" {
name = "myNSG"
location = "Southeast Asia"
resource_group_name = data.azurerm_resource_group.example.name
}
resource "azurerm_network_security_rule" "allow_ssh" {
count = length(var.security_group_rule)
name = var.security_group_rule[count.index].name
direction = "Inbound"
access = "Allow"
protocol = "Tcp"
source_port_range = "*"
source_address_prefix = "*"
destination_address_prefix = "*"
resource_group_name = data.azurerm_resource_group.example.name
network_security_group_name = azurerm_network_security_group.example.name
priority = var.security_group_rule[count.index].priority
destination_port_range = var.security_group_rule[count.index].destination_port_range
}
resource "azurerm_public_ip" "pip" {
count = 2
name = "${count.index}-pip"
resource_group_name = data.azurerm_resource_group.example.name
location = "Southeast Asia"
allocation_method = "Dynamic"
}
resource "azurerm_network_interface" "example" {
count = 2
name = "myNIC1-${count.index}"
location = "Southeast Asia"
resource_group_name = data.azurerm_resource_group.example.name
ip_configuration {
name = "myNIC-${count.index}-config"
subnet_id = azurerm_subnet.example.id
private_ip_address_allocation = "Dynamic"
public_ip_address_id = azurerm_public_ip.pip[count.index].id
}
}
resource "azurerm_network_interface_security_group_association" "example" {
count = 2
network_interface_id = azurerm_network_interface.example[count.index].id
network_security_group_id = azurerm_network_security_group.example.id
}
resource "azurerm_linux_virtual_machine" "example" {
count = 2
name = "myVM-${count.index}"
resource_group_name = data.azurerm_resource_group.example.name
location = "Southeast Asia"
size = "Standard_DS1_v2"
admin_username = "adminuser"
# admin_password = "Password1234!"
# disable_password_authentication = false
admin_ssh_key {
username = "adminuser"
public_key = file("~/.ssh/id_rsa.pub")
}
network_interface_ids = [
azurerm_network_interface.example[count.index].id,
]
os_disk {
caching = "ReadWrite"
storage_account_type = "Standard_LRS"
}
source_image_reference {
publisher = "Canonical"
offer = "UbuntuServer"
sku = "18.04-LTS"
version = "latest"
}
computer_name = "myVM-${count.index}"
provisioner "local-exec" {
command = "echo ${self.public_ip_address} >> inventory"
}
depends_on = [azurerm_public_ip.pip]
}
resource "null_resource" "remoteExecProvisioner" {
triggers = {
always_run = "${timestamp()}"
}
provisioner "local-exec" {
command = "ansible-playbook './ansible-playbook-with-items.yaml' -u adminuser"
}
depends_on = [azurerm_linux_virtual_machine.example]
}
Result :
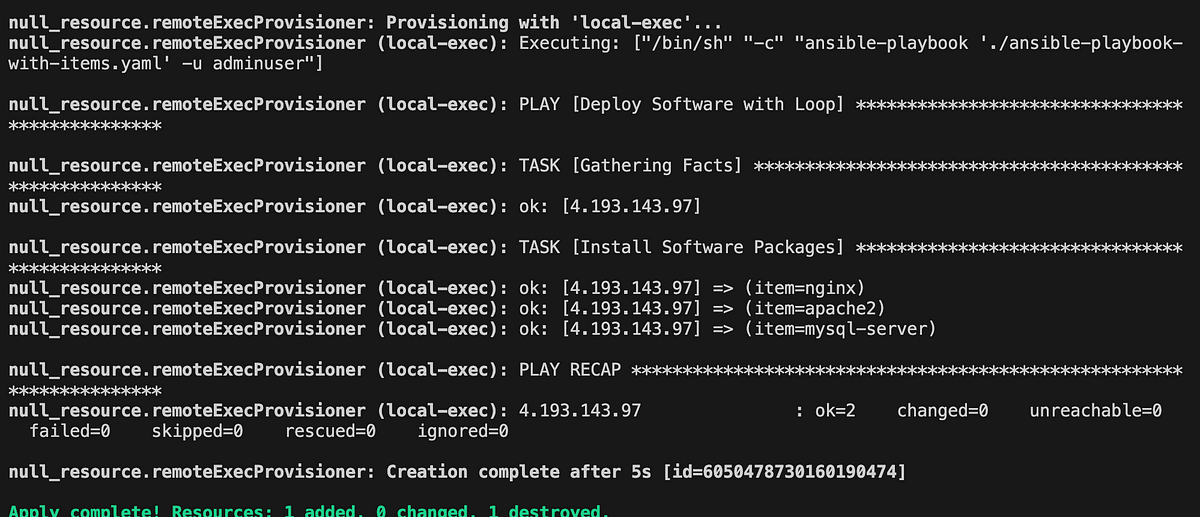